Professional-level analysis by leveraging APIs
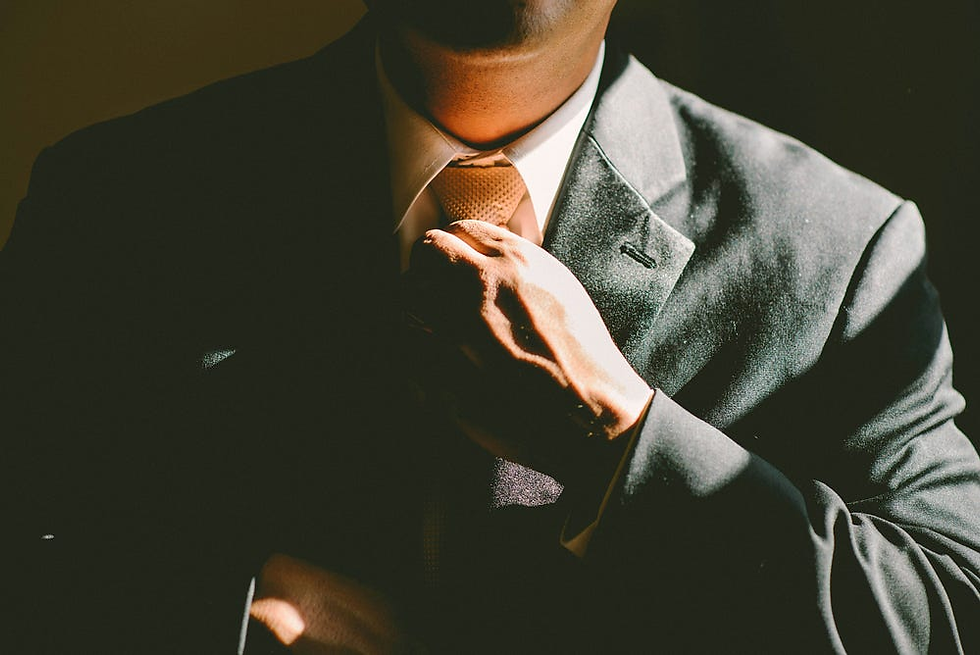
In today’s data-driven financial landscape, accessing and analyzing comprehensive company metrics is crucial for investors and analysts. The Main Street Data API by EODHD offers a powerful tool for accessing over 1,500 company-specific operating metrics, providing a detailed view of a company’s financial health and performance. This blog post will focus on utilizing this API to explore key performance indicators (KPIs) for Apple Inc.
By leveraging the Main Street Data API, we can get into various financial aspects of Apple, such as Revenue by Geography, Revenue by Segment, Gross Margin, Gross Profit, and Operating Expenses. These KPIs provide unique insights into Apple’s operational efficiency and market strategy.
For instance:
Revenue by Geography helps us understand which regions contribute most significantly to Apple’s overall sales.
Revenue by Segment reveals the performance of different product lines or services.
Gross Margin indicates the percentage of revenue that exceeds the cost of goods sold, reflecting Apple’s pricing strategy and production efficiency.
Gross Profit measures the absolute profit after accounting for the cost of goods sold, highlighting Apple’s profitability before operating expenses.
Operating Expenses encompass costs related to running the business, including research and development, marketing, and administrative expenses.
This article will use these KPIs to present Apple’s current financial situation and explore historical trends where applicable. We will also try to understand how Apple maintains its competitive edge in the technology sector through visualizations and detailed explanations.
Get around the Main Street Data API
Before getting into the actual data, we must explore what this API offers us. To do that, let’s see how you ensure which companies are currently supported by this API.
import pandas as pd
import requests
import json
token = 'YOUR API HERE'
url = f'https://eodhd.com/api/mp/mainstreetdata/companies'
query = {"api_token": token}
response = requests.get(url, params=query)
print(f'{len(response.json())} symbols are supported')
At the time of writing this article, it looks like the API supports 424 symbols, and according to EODHD, this list will keep increasing as new symbols will be supported in future updates.
The next step is to explore the KPIs that are offered for each company. For this, you need to check for each company, even though more of the metrics we will investigate in this article are supported by most symbols. Let’s write some code to see which metrics Apple uses!
url = f'https://eodhd.com/api/mp/mainstreetdata/companies/AAPL/kpi'
query = {f"api_token": token}
response = requests.get(url, params=query).json()
df_apple_kpi = pd.DataFrame.from_dict(response)
df_apple_kpi
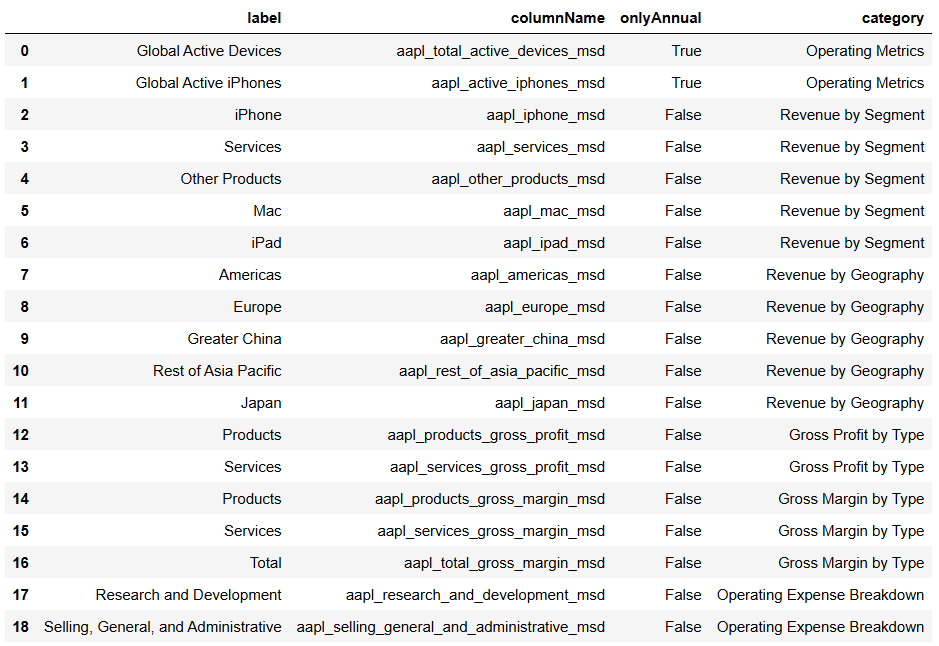
This response will reveal 19 different KPIs from 6 distinct categories. Which are the categories, though?
df_apple_kpi['category'].value_counts()
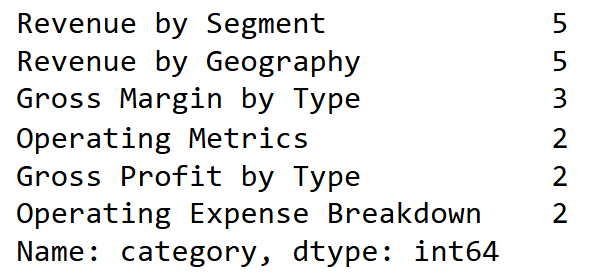
So, let’s deep dive into each category as promised!
Revenue by Geography
We will detail the whole analysis process for each step so you can follow easily and understand why we arrived at the conclusions for each one.
First, we will check the KPIs for this category.
df_apple_kpi[df_apple_kpi['category'] == 'Revenue by Geography']

That is interesting! The way this API is structured, we can easily write a small piece of code to visualize exactly what we are after! We will try to plot the revenue for each geographical area for the last 10 years
start_date = '2014-01-01'
end_date = '2024-12-31'
kpi_list = df_apple_kpi[df_apple_kpi['category'] == 'Revenue by Geography'].to_dict(orient='records')
df = pd.DataFrame()
for kpi in kpi_list:
metric = kpi['columnName']
url = f'https://eodhd.com/api/mp/mainstreetdata/companies/AAPL'
query = {f"api_token": token, "metricName":metric, "startDate":start_date, "endDate":end_date}
response = requests.get(url, params=query).json()
df_m = pd.DataFrame.from_dict(response['metrics'][0]['values']).rename(columns = {"x":"Date", "y":kpi['label']}).drop("valueType", axis=1)
df_m.index = pd.to_datetime(df_m.Date)
df_m.drop("Date", axis=1, inplace=True)
df = pd.merge(df, df_m, left_index=True, right_index=True, how="outer")
df.plot()
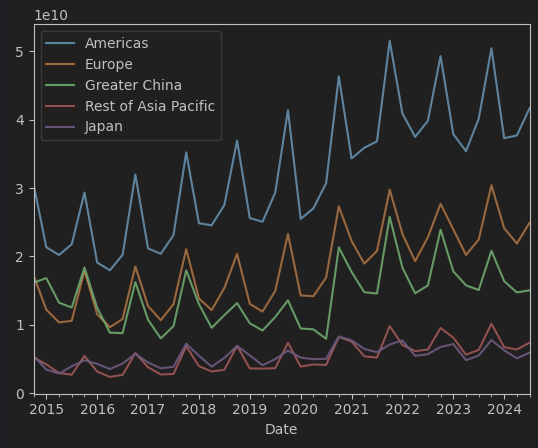
What is interesting here is that we see a lot of picks, and the line is not as smooth as expected. If you check the underlying data, you will realize that in the last quarter of each year, Apple reports significantly more revenue than the other 3 quarters. This is due to Christmas and Black Friday Offers, so from our analysis so far, we can keep that:
Apple is always reporting increased Q4 earnings. However, the market somehow is pre-capitalizing the outcome. If you simply check Apple’s stock price, you will see that at the end of each year, Apple does its yearly highs before the earnings of Q4 are announced!
Now we will smoothen the curve by plotting per year, excluding 2024, since Q4 results have not yet been published.
df_yearly = df[df.index.year < 2024].resample('Y').sum()
df_yearly.plot()
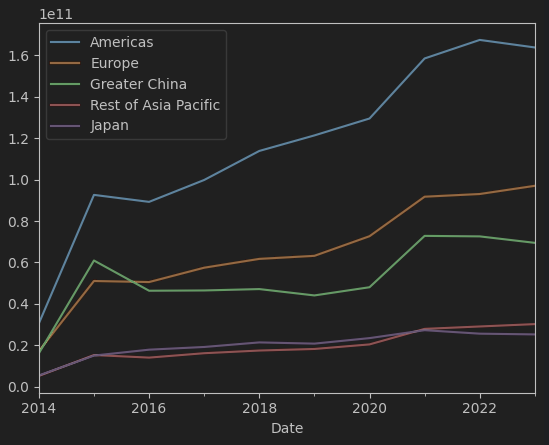
Now things are more clear. We notice that there are no significant changes per geographical area. America is clearly in the lead, with Europe in second place. Japan and the rest of Asia Pacific are very close without a clear over the years winner. And to see the latest numbers in percentages, it is a simple two-liner code:
df_percentage = df.div(df.sum(axis=1), axis=0) * 100
df_percentage.tail(1)

Americas has 43.8% of the global revenue and Europe 26.2%. Greater China had a surprising 15.8%, while the rest had 14%.
What we can keep from these numbers is that potential disruptions in the Greater China area will have a significant impact on Apple’s revenue.
Revenue by Segment
Let’s move now to analyze the revenue by segment. We will see how this KPI is described in EODHD Main Street Data API.
df_apple_kpi[df_apple_kpi['category'] == 'Revenue by Segment']
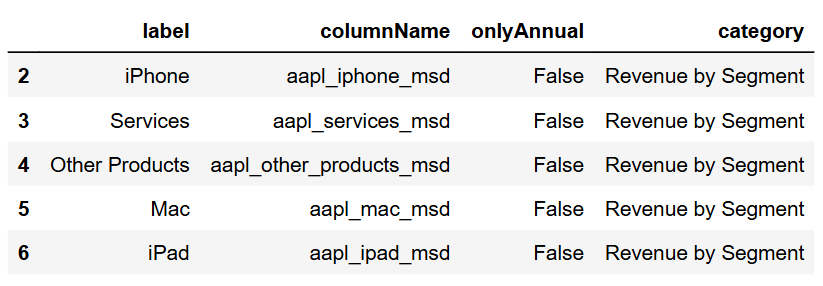
As expected! The breakdown makes complete sense, separating the revenue per iPhones, Macs, iPads, and other products and services. As before, we will plot the revenue per segment for the last 10 years with a similar code. As we realized before, it is better to calculate per year, excluding 2024.
start_date = '2014-01-01'
end_date = '2024-12-31'
kpi_list = df_apple_kpi[df_apple_kpi['category'] == 'Revenue by Segment'].to_dict(orient='records')
df = pd.DataFrame()
for kpi in kpi_list:
metric = kpi['columnName']
# print(kpi['label'])
url = f'https://eodhd.com/api/mp/mainstreetdata/companies/AAPL'
query = {f"api_token": token, "metricName":metric, "startDate":start_date, "endDate":end_date}
response = requests.get(url, params=query).json()
# print(response['metrics'][0]['values'])
df_m = pd.DataFrame.from_dict(response['metrics'][0]['values']).rename(columns = {"x":"Date", "y":kpi['label']}).drop("valueType", axis=1)
df_m.index = pd.to_datetime(df_m.Date)
df_m.drop("Date", axis=1, inplace=True)
df = pd.merge(df, df_m, left_index=True, right_index=True, how="outer")
df_yearly = df.resample('Y').sum()
df_yearly.plot()

It was expected to see that the iPhone is significantly higher, so before starting to talk about it, better to see also the latest weights
df_percentage = df.div(df.sum(axis=1), axis=0) * 100
df_percentage.tail(1)

Personally, from the above, I would keep 2 things:
First is that iPhone is constantly increasing each share on Apple’s revenue, and it is already close to 50% to the total global revenue.
However, the iPhone’s dominance in the revenue area might be obvious; what is interesting is the linearly steady increase in services!
This means that services like Apple TV, Apple Store, or even Apple Pay are constantly increasing their share of the revenue, making it a significant factor in the company’s profits.
Gross Margin and Gross Profit
Considering the increase in services in Apple’s total revenue, it makes sense to see the gross margin of the services and try to find some reasoning. So, let’s check the Gross Margin KPIs.
df_apple_kpi[df_apple_kpi['category'] == 'Gross Margin by Type']
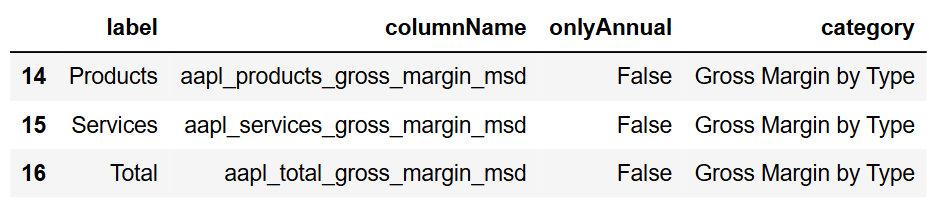
Those KPI’s are smartly separated into products and services, as well as the total.
start_date = '2014-01-01'
end_date = '2024-12-31'
kpi_list = df_apple_kpi[df_apple_kpi['category'] == 'Gross Margin by Type'].to_dict(orient='records')
df = pd.DataFrame()
for kpi in kpi_list:
metric = kpi['columnName']
# print(kpi['label'])
url = f'https://eodhd.com/api/mp/mainstreetdata/companies/AAPL'
query = {f"api_token": token, "metricName":metric, "startDate":start_date, "endDate":end_date}
response = requests.get(url, params=query).json()
# print(response['metrics'][0]['values'])
df_m = pd.DataFrame.from_dict(response['metrics'][0]['values']).rename(columns = {"x":"Date", "y":kpi['label']}).drop("valueType", axis=1)
df_m.index = pd.to_datetime(df_m.Date)
df_m.drop("Date", axis=1, inplace=True)
df = pd.merge(df, df_m, left_index=True, right_index=True, how="outer")
df.plot()
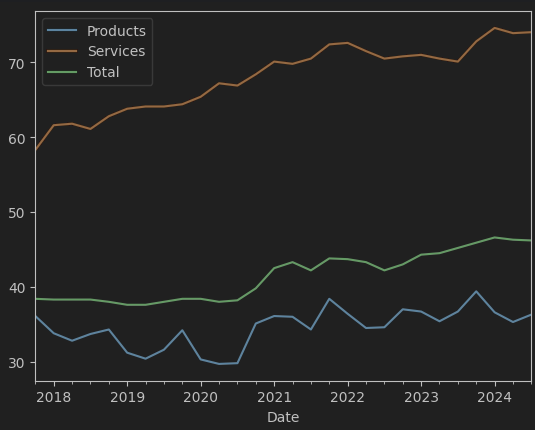
That explains it all! The services constantly increase their Gross Margin from around 60% in 2014 to around 75% in 2024. A gross margin of 75% in Services means that for every $100 Apple earns from its Services segment, $75 is retained as gross profit after covering the direct costs associated with delivering those services. This high margin reflects the low cost of goods sold (COGS) for services like the App Store, iCloud, Apple Music, and Apple Pay, which primarily involve software, licensing, or digital content rather than physical products.
No wonder Apple is constantly increasing its revenues in that area!
But what about Gross Profits?
start_date = '2014-01-01'
end_date = '2024-12-31'
kpi_list = df_apple_kpi[df_apple_kpi['category'] == 'Gross Profit by Type'].to_dict(orient='records')
df = pd.DataFrame()
for kpi in kpi_list:
metric = kpi['columnName']
url = f'https://eodhd.com/api/mp/mainstreetdata/companies/AAPL'
query = {f"api_token": token, "metricName":metric, "startDate":start_date, "endDate":end_date}
response = requests.get(url, params=query).json()
# print(response['metrics'][0]['values'])
df_m = pd.DataFrame.from_dict(response['metrics'][0]['values']).rename(columns = {"x":"Date", "y":kpi['label']}).drop("valueType", axis=1)
df_m.index = pd.to_datetime(df_m.Date)
df_m.drop("Date", axis=1, inplace=True)
df = pd.merge(df, df_m, left_index=True, right_index=True, how="outer")
df_yearly = df[df.index.year < 2024].resample('Y').sum()
df_yearly.plot()
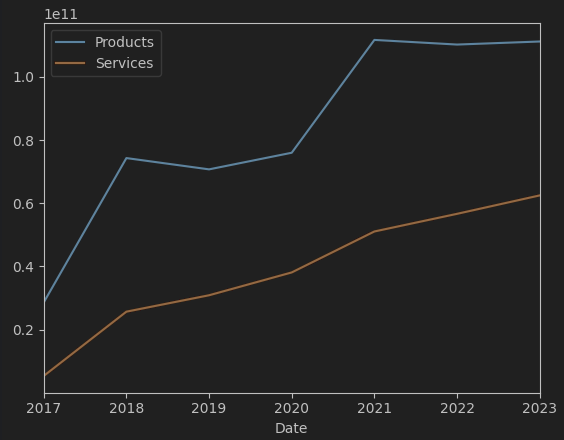
The product’s Gross Profit is higher, even though the Gross Margin is significantly less. This is because products like iPhones, Macs, etc., are still the leader in their sales, and regardless of the smaller margins, they are still the largest part of Apple’s profits.
Operating Expenses Breakdown
Besides the above KPIs directly connected with Apple’s revenue streams, it is good to get some answers regarding Apple’s expenses.
df_apple_kpi[df_apple_kpi['category'] == 'Operating Expense Breakdown']

We can see that the API offers us a breakdown between research and development compared to general sales and admin.
start_date = '2010-01-01'
end_date = '2024-12-31'
kpi_list = df_apple_kpi[df_apple_kpi['category'] == 'Operating Expense Breakdown'].to_dict(orient='records')
df = pd.DataFrame()
for kpi in kpi_list:
metric = kpi['columnName']
url = f'https://eodhd.com/api/mp/mainstreetdata/companies/AAPL'
query = {f"api_token": token, "metricName":metric, "startDate":start_date, "endDate":end_date}
response = requests.get(url, params=query).json()
# print(response['metrics'])
df_m = pd.DataFrame.from_dict(response['metrics'][0]['values']).rename(columns = {"x":"Date", "y":kpi['label']}).drop("valueType", axis=1)
df_m.index = pd.to_datetime(df_m.Date)
df_m.drop("Date", axis=1, inplace=True)
df = pd.merge(df, df_m, left_index=True, right_index=True, how="outer")
df.plot()
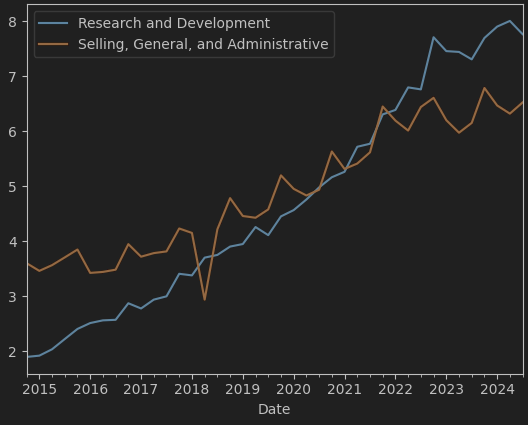
I have to say that this is a very interesting plot. We can understand from this one that in the last decade till 2020, Apple focused on sales. Since that year, there has been a shift in research and development. Actually, this is not a once-off, but research and development incline on expenses is more stip than sales and admin. It seems that Apple is getting more focused on creating new products (or updating iPhones ;) than actually managing the sales.
Conclusions
Let’s recap what we found for Apple using EODHD Main Street Data API:
Revenue by Geography: Apple’s revenue peaks in Q4 due to holiday spending, with Americas leading, followed by Europe. Greater China’s significant share suggests potential revenue impact from regional disruptions.
Revenue by Segment: iPhone dominates revenue, nearing 50% of the total. Services steadily increase, highlighting their growing importance in Apple’s revenue mix.
Gross Margin and Profit: Services boast a high gross margin of 75%, contributing significantly to profits despite lower revenue than products. Products like iPhones still lead in gross profit due to higher sales volume.
Operating Expenses: Recent trends indicate a strategic shift towards increased investment in Research and Development over sales and administrative expenses, signaling a focus on innovation.
The data are proving it that Apple knows exactly what it’s doing — dangling the iPhone as the carrot to draw you in, then locking you into its ecosystem with services as the stick. After all, as they say, ‘Why sell a product once when you can sell a subscription forever?
Comments